AM0 نشر 31 أكتوبر 2020 أرسل تقرير نشر 31 أكتوبر 2020 كيف انشئ برنامج يستخدم دالة تسمى CountSpaces()تستقبل جملة نصية من المستخدم وتطبع عدد المسافات الفارغة " " في هذه الجملة ؟ يعني زي كذا 1 اقتباس
0 Wael Aljamal نشر 31 أكتوبر 2020 أرسل تقرير نشر 31 أكتوبر 2020 مرحبا أنور، يمكنك استخدام المثال التالي: using System; public class funcexer4 { public static int SpaceCount(string str) { int spcctr=0; string str1; for (int i = 0;i < str.Length;i++) { str1 = str.Substring(i,1); if (str1 == " ") spcctr++; } return spcctr; } public static void Main() { string str2; Console.Write("\n\nFunction to count number of spaces in a string :\n"); Console.Write("----------------------------------------------------\n"); Console.Write("Please input a string : "); str2 = Console.ReadLine(); Console.WriteLine("\""+str2+"\""+" contains {0} spaces", SpaceCount(str2) ); } } و الخرج سيكون هكذا: Function to count number of spaces in a string : ---------------------------------------------------- Please input a string : w3resource is a tutorial "w3resource is a tutorial" contains 3 spaces العمل: نمر على السلسة النصية و نطابق كل حرف منها مع محرف الفراغ " " و نزيد قيمة العداد اقتباس
0 ماجد قطوسة نشر 31 أكتوبر 2020 أرسل تقرير نشر 31 أكتوبر 2020 مرحبا بك . أرفق لك كود به طريقتين لتنفذ المطلوب . using System; class Program { static void Main() { string input = "Dot Net Perls website"; // // Check each character in the string using for-loop. // int spaces1 = 0; for (int i = 0; i < input.Length; i++) { if (input[i] == ' ') { spaces1++; } } // // BAD: Check each character in the string with ToString calls. // int spaces2 = 0; for (int i = 0; i < input.Length; i++) { if (input[i].ToString() == " ") // NO { spaces2++; } } // // Write results. // Console.WriteLine(spaces1); Console.WriteLine(spaces2); } } النتائج : 3 3 شكراً لك اقتباس
0 عزام عبد الحافظ نشر 31 أكتوبر 2020 أرسل تقرير نشر 31 أكتوبر 2020 مرحبًا @Anwar Mohammed, يمكنك عمل ذلك بهذه الشيفرة : public class Program { // هنا دالة public static void CountSpaces(string txt) { // متغير لحسب فرغات النص int count = 0; // عملية التكرار تقوم بمرور على جميع أحرف النص للبحث عن الفرغات for( int i = 0; i < txt.Length; i++) { // count اذا كان الحرف مساحة فارغة نقوم بزيادة عدد المتغير if(txt[i] == ' ') { count++; } } Console.WriteLine("Your text has " + Convert.ToString(count) + " spaces"); } public static void Main(string[] args) { Console.WriteLine("Please enter your text : "); //txt نقوم بتخزين نص المستخدم في متفير string txt = Console.ReadLine(); // الدالة CountSpaces(txt); } } اقتباس
0 Omar Haddad2 نشر 31 أكتوبر 2020 أرسل تقرير نشر 31 أكتوبر 2020 يمكن الحل من خلال إستخدام حلقة للمرور على النص خطوة بخطوة دالة substring وذالك لتجزئة النصة ومقارنة الحرف الواحد أو ب فراغ يمكن تنفيذ الحل من هنا using System; public class Space { public static int SpaceCount(string str) { //المتغير الخاص بالعد int spcount=0 // النص الذي سيتم عد المساحات الفارغة به string input1; //حلقة للمرور على النص الذي يتم إدخاله for (int i = 0;i < str.Length;i++) { //تستخدم هذه الدالة لتجزئة النصوص input1 = str.Substring(i,1); if (input1 == " ") //إضافة 1 للمتغير الخاص بالعد spcount++; } return spcount; } public static void Main() { //تنفيذ string input2; Console.Write("Please input a string : "); input2 = Console.ReadLine(); Console.WriteLine("\""+input2+"\""+" contains {0} spaces", SpaceCount(input2) ); } } اقتباس
0 ayoubridouani نشر 31 أكتوبر 2020 أرسل تقرير نشر 31 أكتوبر 2020 مرحبا, يمكنك حساب عدد الفراغات في سطر واحد برمجي لا أكثر بالطريقة التالية: using System; public class Ayoub { public static void Main() { Console.Write("Please enter your string : "); string _string = Console.ReadLine(); Console.WriteLine(_string.Split().Length - 1); } } حيث هنا قمت بتقسيم string لمصفوفة ب sep مساوٍ ل space (يكون إفتراضيا) بعدها حسبت عدد العناصر. اقتباس
0 سمير عبود نشر 31 أكتوبر 2020 أرسل تقرير نشر 31 أكتوبر 2020 مرحباً @AM0 بإمكانك إستخدام LINQ لحساب عدد تكرار محرف مُعين في سلسلة نصية ما و ذلك من خلال: static int countChars(string mystring, char c) { return mystring.Count(s => s == c); // العد يكون على أساس تساوي المحرف الحالي مع المحرف المطلوب } و هذا البرنامج ككل: using System; using System.Linq; // لإستخدام الميثود Count class HelloWorld { static void Main() { Console.Write("Please enter your string : "); string mystring = Console.ReadLine(); Console.WriteLine(countChars(mystring, ' ')); } static int countChars(string mystring, char c) { return mystring.Count(s => s == c); // العد يكون على أساس تساوي المحرف الحالي مع المحرف المطلوب } } كما يُمكنك تجربة المثال من: هنا بالتوفيق. اقتباس
السؤال
AM0
كيف انشئ برنامج يستخدم دالة تسمى CountSpaces()تستقبل جملة نصية من المستخدم وتطبع عدد المسافات الفارغة " " في هذه الجملة ؟ يعني زي كذا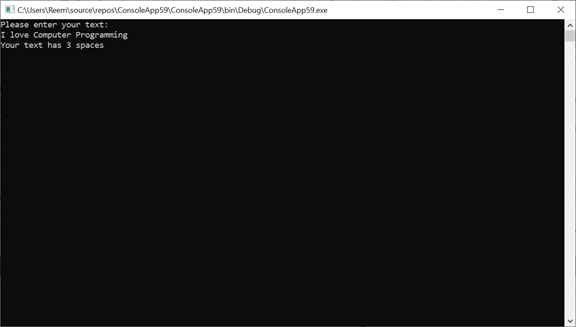
6 أجوبة على هذا السؤال
Recommended Posts
انضم إلى النقاش
يمكنك أن تنشر الآن وتسجل لاحقًا. إذا كان لديك حساب، فسجل الدخول الآن لتنشر باسم حسابك.