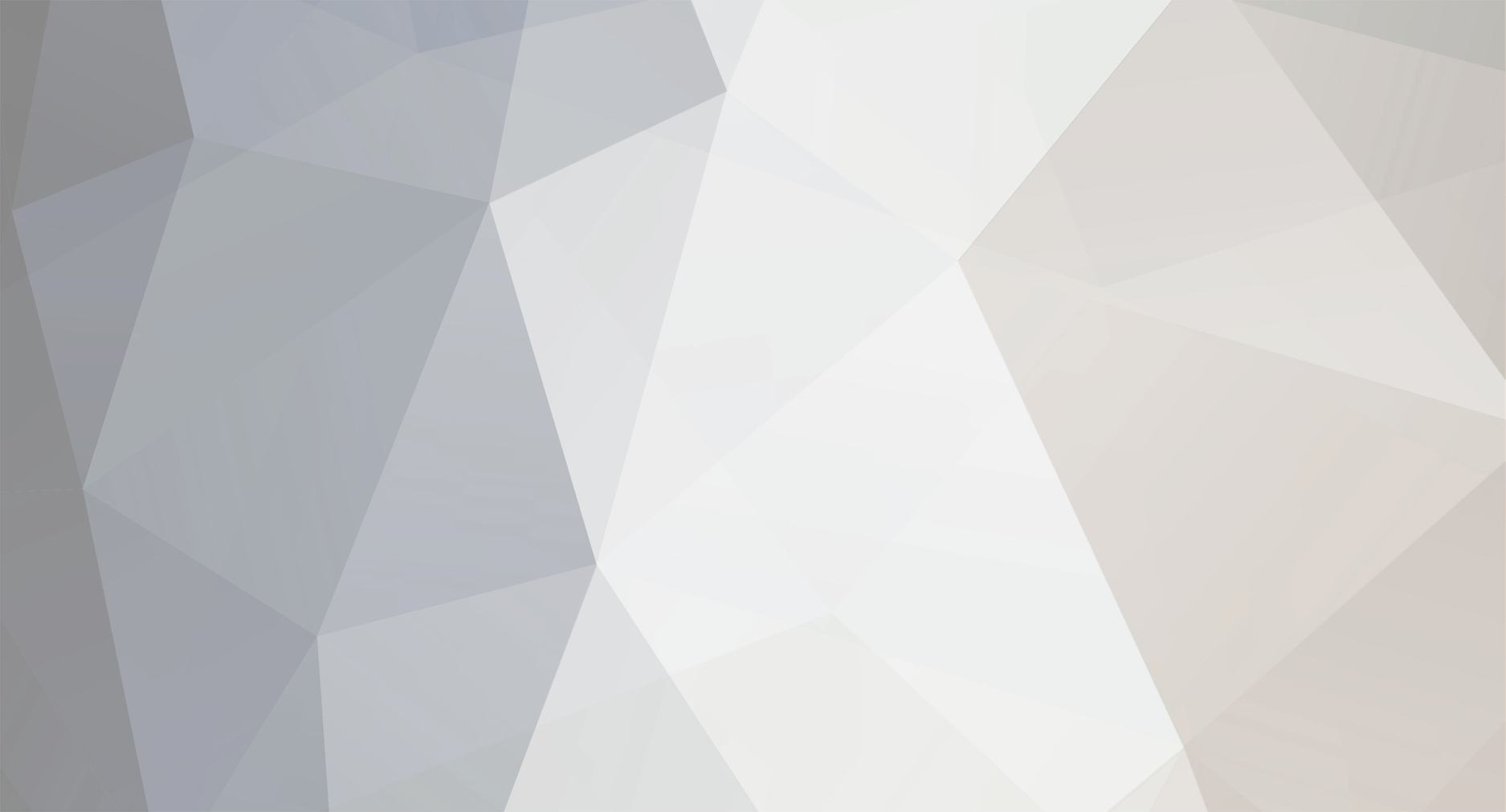
Enas Wawy
-
المساهمات
2 -
تاريخ الانضمام
-
تاريخ آخر زيارة
نوع المحتوى
ريادة الأعمال
البرمجة
التصميم
DevOps
التسويق والمبيعات
العمل الحر
البرامج والتطبيقات
آخر التحديثات
قصص نجاح
أسئلة وأجوبة
كتب
دورات
أجوبة بواسطة Enas Wawy
-
-
بدي حدا يحللي هاد السؤال ضروري حاولت ما زبط معي؟
1- قم بإنشاء صنف (Dealer) وفق التالي:
a. المتغيرات:
i. الاسم -:Name محمي من نوع نص.
b. الدوال:
i. بناء يستقبل معامل ويقوم بوضع قيم ابتدائية للاسم. القيمة الافتراضية للمعامل هي "Unknown".
ii. دالة عامة ترجع قيمة الاسم.
iii. دالة عامة تستقبل معامل وتغير من خلاله قيمة الاسم.2- قم بإنشاء صنف (PlayStation) وفق التالي:
a. المتغيرات:
i. الرقم - :No خاص من نوع رقم صحيح.
ii. السعر - :price محمي رقمي عشري.
b. الدوال:
i. بناء يقوم بقراءة قيم للمتغيرات من خلال لوحة المفاتيح.
ii. دالة عامة ترجع قيمة السعر.
iii. دالة عامة تستقبل معامل وتغير من خلاله قيمة السعر.3- قم بإنشاء صنف (Invoice) يرث من الصنف (Dealer) وراثة عامة وفق التالي:
a. المتغيرات:
i. :PlayStations عامة مصفوفة مكونة من 5 كائنات (PlayStation).
ii. Total: خاص رقمي عشري.
b. الدوال:
i. ReadPrices(): عامة وتقوم بقراءة قيم عناصر المصفوفة (PlayStations) .
ii. GetTotal(): عامة وتحسب مجموع الاسعار للمصفوفة وتخزينه داخل المتغير (Total) ثم تقوم بارجاعه.
iii. GetMax(): عامة ترجع اعلى الاسعار للمصفوفة.
iv. بناء يقوم بحساب مجموع الاسعار للمصفوفة وتخزينه داخل المتغير (Total).
v. :Print() عامة وتطبع على الشاشة جميع المتغيرات المحلية والموروثة.
vi. بين مفهوم إعادة التحميل مستخدما الدالة (Print) باستقبالها اسم ملف وكتابة جميع المتغيرات المحلية والموروثة داخل الملف.4- الدالة الرئيسية:
a. انشاء مصفوفة كائنات ديناميكيا من نوع (Invoice) تتكون من أي عدد من عندك.
b. استدعاء جميع الدوال الخاصة والموروثة بالكائن السابق.
(مساعدة في كود c++)
في أسئلة البرمجة
نشر